Browse Source
What are your thoughts on parameterizing the install script for local install? I know this seems weird but it means that I don't have to rely on developers having any tools already installed except bash and some way to edit the files. Here is an actual example script setup I would use. Here is an example local install script `installLocal.sh` ```bash #!/usr/bin/env bash DESIRED_NODE_VERSION=v$(cat package.json | grep -Ei "\"node\"" | grep -Eoi "[0-9]+\.[0-9]+\.[0-9]+") export FNM_DIR="$(pwd)/.fnm" if [ ! -d "$FNM_DIR" ]; then curl 'https://raw.githubusercontent.com/Schniz/fnm/master/.ci/install.sh' | INSTALL_DIR=`pwd` bash fi set -e NODE_DIR="$FNM_DIR/node-versions/$DESIRED_NODE_VERSION/installation/bin" export PATH=$FNM_DIR:$NODE_DIR:$PATH #TODO: support fish eval `fnm env --multi` if [ ! -f "$NODE_DIR/node" ]; then fnm install $DESIRED_NODE_VERSION fi sumOfPackageJson() { cat package.json | md5sum | cut -d' ' -f1 } if [ ! -f ".packageRevision" ] || [ "$(cat .packageRevision)" != "$(sumOfPackageJson)" ]; then sumOfPackageJson > .packageRevision npm install fi ``` then in script called `nodew` ```bash #!/usr/bin/env bash cd "$( dirname "${BASH_SOURCE[0]}" )" source ./installLocal.sh node "$@" ``` and `npmw` ```bash #!/usr/bin/env bash cd "$( dirname "${BASH_SOURCE[0]}" )" source ./installLocal.sh npm "$@" ``` and for completeness sake I usually go one step further and do an `appw` ```bash #!/usr/bin/env bash cd "$( dirname "${BASH_SOURCE[0]}" )" source ./installLocal.sh node ./app/index.js "$@" ```remotes/origin/add-simple-redirecting-site
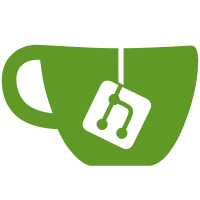
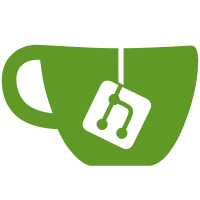
2 changed files with 50 additions and 4 deletions
Loading…
Reference in new issue