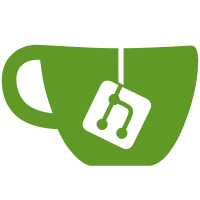
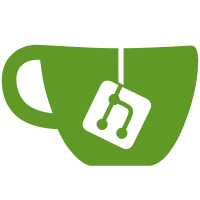
9 changed files with 699 additions and 140 deletions
@ -0,0 +1,152 @@
@@ -0,0 +1,152 @@
|
||||
#!/usr/bin/env node
|
||||
|
||||
/// @ts-check
|
||||
|
||||
const execa = require("execa"); |
||||
const path = require("path"); |
||||
const fs = require("fs"); |
||||
const cmd = require("cmd-ts"); |
||||
const cmdFs = require("cmd-ts/dist/cjs/batteries/fs"); |
||||
|
||||
const FnmBinaryPath = { |
||||
...cmdFs.ExistingPath, |
||||
defaultValue() { |
||||
const target = path.join(__dirname, "../target/debug/fnm"); |
||||
if (!fs.existsSync(target)) { |
||||
throw new Error( |
||||
"Can't find debug target, please run `cargo build` or provide a specific binary path" |
||||
); |
||||
} |
||||
return target; |
||||
}, |
||||
}; |
||||
|
||||
const command = cmd.command({ |
||||
name: "print-command-docs", |
||||
description: "prints the docs/command.md file with updated contents", |
||||
args: { |
||||
checkForDirty: cmd.flag({ |
||||
long: "check", |
||||
description: `Check that file was not changed`, |
||||
}), |
||||
fnmPath: cmd.option({ |
||||
long: "binary-path", |
||||
description: "the fnm binary path", |
||||
type: FnmBinaryPath, |
||||
}), |
||||
}, |
||||
async handler({ checkForDirty, fnmPath }) { |
||||
const targetFile = path.join(__dirname, "../docs/commands.md"); |
||||
await main(targetFile, fnmPath); |
||||
if (checkForDirty) { |
||||
const gitStatus = await checkGitStatus(targetFile); |
||||
if (gitStatus.state === "dirty") { |
||||
process.exitCode = 1; |
||||
console.error( |
||||
"The file has changed. Please re-run `yarn generate-command-docs`." |
||||
); |
||||
console.error(`hint: The following diff was found:`); |
||||
console.error(); |
||||
console.error(gitStatus.diff); |
||||
} |
||||
} |
||||
}, |
||||
}); |
||||
|
||||
cmd.run(cmd.binary(command), process.argv).catch((err) => { |
||||
console.error(err); |
||||
process.exitCode = process.exitCode || 1; |
||||
}); |
||||
|
||||
/** |
||||
* @param {string} targetFile |
||||
* @param {string} fnmPath |
||||
* @returns {Promise<void>} |
||||
*/ |
||||
async function main(targetFile, fnmPath) { |
||||
const stream = fs.createWriteStream(targetFile); |
||||
|
||||
const { subcommands, text: mainText } = await getCommandHelp(fnmPath); |
||||
|
||||
await write(stream, line(`fnm`, mainText)); |
||||
|
||||
for (const subcommand of subcommands) { |
||||
const { text: subcommandText } = await getCommandHelp(fnmPath, subcommand); |
||||
await write(stream, "\n" + line(`fnm ${subcommand}`, subcommandText)); |
||||
} |
||||
|
||||
stream.close(); |
||||
|
||||
await execa(`yarn`, ["prettier", "--write", targetFile]); |
||||
} |
||||
|
||||
/** |
||||
* @param {import('stream').Writable} stream |
||||
* @param {string} content |
||||
* @returns {Promise<void>} |
||||
*/ |
||||
function write(stream, content) { |
||||
return new Promise((resolve, reject) => { |
||||
stream.write(content, (err) => (err ? reject(err) : resolve())); |
||||
}); |
||||
} |
||||
|
||||
function line(cmd, text) { |
||||
const cmdCode = "`" + cmd + "`"; |
||||
const textCode = "```\n" + text + "\n```"; |
||||
return `# ${cmdCode}\n${textCode}`; |
||||
} |
||||
|
||||
/** |
||||
* @param {string} fnmPath |
||||
* @param {string} [command] |
||||
* @returns {Promise<{ subcommands: string[], text: string }>} |
||||
*/ |
||||
async function getCommandHelp(fnmPath, command) { |
||||
const cmdArg = command ? [command] : []; |
||||
const result = await run(fnmPath, [...cmdArg, "--help"]); |
||||
const text = result.stdout; |
||||
const rows = text.split("\n"); |
||||
const headerIndex = rows.findIndex((x) => x.includes("SUBCOMMANDS")); |
||||
/** @type {string[]} */ |
||||
const subcommands = []; |
||||
for (const row of rows.slice(headerIndex + 1)) { |
||||
const matched = row.match(/^\s{4}(\w+)/); |
||||
if (!matched) break; |
||||
subcommands.push(matched[1]); |
||||
} |
||||
return { |
||||
subcommands, |
||||
text, |
||||
}; |
||||
} |
||||
|
||||
/** |
||||
* @param {string[]} args |
||||
* @returns {import('execa').ExecaChildProcess<string>} |
||||
*/ |
||||
function run(fnmPath, args) { |
||||
return execa(fnmPath, args, { |
||||
reject: false, |
||||
stdout: "pipe", |
||||
stderr: "pipe", |
||||
}); |
||||
} |
||||
|
||||
/** |
||||
* @param {string} targetFile |
||||
* @returns {Promise<{ state: "dirty", diff: string } | { state: "clean" }>} |
||||
*/ |
||||
async function checkGitStatus(targetFile) { |
||||
const { stdout, exitCode } = await execa( |
||||
`git`, |
||||
["diff", "--color", "--exit-code", targetFile], |
||||
{ |
||||
reject: false, |
||||
} |
||||
); |
||||
if (exitCode === 0) { |
||||
return { state: "clean" }; |
||||
} |
||||
return { state: "dirty", diff: stdout }; |
||||
} |
@ -0,0 +1,402 @@
@@ -0,0 +1,402 @@
|
||||
# `fnm` |
||||
|
||||
``` |
||||
fnm 1.24.0 |
||||
A fast and simple Node.js manager |
||||
|
||||
USAGE: |
||||
fnm [OPTIONS] <SUBCOMMAND> |
||||
|
||||
FLAGS: |
||||
-h, --help |
||||
Prints help information |
||||
|
||||
-V, --version |
||||
Prints version information |
||||
|
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> |
||||
The root directory of fnm installations [env: FNM_DIR] |
||||
|
||||
--log-level <log-level> |
||||
The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
|
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
|
||||
SUBCOMMANDS: |
||||
alias Alias a version to a common name |
||||
completions Print shell completions to stdout |
||||
current Print the current Node.js version |
||||
default Set a version as the default version |
||||
env Print and set up required environment variables for fnm |
||||
exec Run a command within fnm context |
||||
help Prints this message or the help of the given subcommand(s) |
||||
install Install a new Node.js version |
||||
list List all locally installed Node.js versions [aliases: ls] |
||||
list-remote List all remote Node.js versions [aliases: ls-remote] |
||||
uninstall Uninstall a Node.js version |
||||
use Change Node.js version |
||||
``` |
||||
|
||||
# `fnm alias` |
||||
|
||||
``` |
||||
fnm-alias 1.24.0 |
||||
Alias a version to a common name |
||||
|
||||
USAGE: |
||||
fnm alias [OPTIONS] <to-version> <name> |
||||
|
||||
FLAGS: |
||||
-h, --help Prints help information |
||||
-V, --version Prints version information |
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> The root directory of fnm installations [env: FNM_DIR] |
||||
--log-level <log-level> The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
|
||||
ARGS: |
||||
<to-version> |
||||
<name> |
||||
``` |
||||
|
||||
# `fnm completions` |
||||
|
||||
``` |
||||
fnm-completions 1.24.0 |
||||
Print shell completions to stdout |
||||
|
||||
USAGE: |
||||
fnm completions [OPTIONS] |
||||
|
||||
FLAGS: |
||||
-h, --help Prints help information |
||||
-V, --version Prints version information |
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> The root directory of fnm installations [env: FNM_DIR] |
||||
--log-level <log-level> The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
--shell <shell> |
||||
The shell syntax to use. Infers when missing [possible values: zsh, bash, fish, powershell, elvish] |
||||
|
||||
``` |
||||
|
||||
# `fnm current` |
||||
|
||||
``` |
||||
fnm-current 1.24.0 |
||||
Print the current Node.js version |
||||
|
||||
USAGE: |
||||
fnm current [OPTIONS] |
||||
|
||||
FLAGS: |
||||
-h, --help Prints help information |
||||
-V, --version Prints version information |
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> The root directory of fnm installations [env: FNM_DIR] |
||||
--log-level <log-level> The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
``` |
||||
|
||||
# `fnm default` |
||||
|
||||
``` |
||||
fnm-default 1.24.0 |
||||
Set a version as the default version |
||||
|
||||
This is a shorthand for `fnm alias VERSION default` |
||||
|
||||
USAGE: |
||||
fnm default [OPTIONS] <version> |
||||
|
||||
FLAGS: |
||||
-h, --help |
||||
Prints help information |
||||
|
||||
-V, --version |
||||
Prints version information |
||||
|
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> |
||||
The root directory of fnm installations [env: FNM_DIR] |
||||
|
||||
--log-level <log-level> |
||||
The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
|
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
|
||||
ARGS: |
||||
<version> |
||||
|
||||
|
||||
``` |
||||
|
||||
# `fnm env` |
||||
|
||||
``` |
||||
fnm-env 1.24.0 |
||||
Print and set up required environment variables for fnm |
||||
|
||||
This command generates a series of shell commands that should be evaluated by your shell to create a fnm-ready |
||||
environment. |
||||
|
||||
Each shell has its own syntax of evaluating a dynamic expression. For example, evaluating fnm on Bash and Zsh would look |
||||
like `eval "$(fnm env)"`. In Fish, evaluating would look like `fnm env | source` |
||||
|
||||
USAGE: |
||||
fnm env [FLAGS] [OPTIONS] |
||||
|
||||
FLAGS: |
||||
-h, --help |
||||
Prints help information |
||||
|
||||
--use-on-cd |
||||
Print the script to change Node versions every directory change |
||||
|
||||
-V, --version |
||||
Prints version information |
||||
|
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> |
||||
The root directory of fnm installations [env: FNM_DIR] |
||||
|
||||
--log-level <log-level> |
||||
The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
|
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
--shell <shell> |
||||
The shell syntax to use. Infers when missing [possible values: bash, zsh, fish, powershell] |
||||
|
||||
``` |
||||
|
||||
# `fnm exec` |
||||
|
||||
``` |
||||
fnm-exec 1.24.0 |
||||
Run a command within fnm context |
||||
|
||||
Example: |
||||
-------- |
||||
fnm exec --using=v12.0.0 node --version |
||||
=> v12.0.0 |
||||
|
||||
USAGE: |
||||
fnm exec [OPTIONS] [arguments]... |
||||
|
||||
FLAGS: |
||||
-h, --help |
||||
Prints help information |
||||
|
||||
-V, --version |
||||
Prints version information |
||||
|
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> |
||||
The root directory of fnm installations [env: FNM_DIR] |
||||
|
||||
--log-level <log-level> |
||||
The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
|
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
--using <version> |
||||
Either an explicit version, or a filename with the version written in it |
||||
|
||||
|
||||
ARGS: |
||||
<arguments>... |
||||
The command to run |
||||
|
||||
``` |
||||
|
||||
# `fnm help` |
||||
|
||||
``` |
||||
|
||||
``` |
||||
|
||||
# `fnm install` |
||||
|
||||
``` |
||||
fnm-install 1.24.0 |
||||
Install a new Node.js version |
||||
|
||||
USAGE: |
||||
fnm install [FLAGS] [OPTIONS] [version] |
||||
|
||||
FLAGS: |
||||
-h, --help Prints help information |
||||
--lts Install latest LTS |
||||
-V, --version Prints version information |
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> The root directory of fnm installations [env: FNM_DIR] |
||||
--log-level <log-level> The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
|
||||
ARGS: |
||||
<version> A version string. Can be a partial semver or a LTS version name by the format lts/NAME |
||||
``` |
||||
|
||||
# `fnm list` |
||||
|
||||
``` |
||||
fnm-list 1.24.0 |
||||
List all locally installed Node.js versions |
||||
|
||||
USAGE: |
||||
fnm list [OPTIONS] |
||||
|
||||
FLAGS: |
||||
-h, --help Prints help information |
||||
-V, --version Prints version information |
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> The root directory of fnm installations [env: FNM_DIR] |
||||
--log-level <log-level> The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
``` |
||||
|
||||
# `fnm list` |
||||
|
||||
``` |
||||
fnm-list 1.24.0 |
||||
List all locally installed Node.js versions |
||||
|
||||
USAGE: |
||||
fnm list [OPTIONS] |
||||
|
||||
FLAGS: |
||||
-h, --help Prints help information |
||||
-V, --version Prints version information |
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> The root directory of fnm installations [env: FNM_DIR] |
||||
--log-level <log-level> The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
``` |
||||
|
||||
# `fnm uninstall` |
||||
|
||||
``` |
||||
fnm-uninstall 1.24.0 |
||||
Uninstall a Node.js version |
||||
|
||||
> Warning: when providing an alias, it will remove the Node version the alias is pointing to, along with the other |
||||
aliases that point to the same version. |
||||
|
||||
USAGE: |
||||
fnm uninstall [OPTIONS] [version] |
||||
|
||||
FLAGS: |
||||
-h, --help |
||||
Prints help information |
||||
|
||||
-V, --version |
||||
Prints version information |
||||
|
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> |
||||
The root directory of fnm installations [env: FNM_DIR] |
||||
|
||||
--log-level <log-level> |
||||
The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
|
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
|
||||
ARGS: |
||||
<version> |
||||
|
||||
|
||||
``` |
||||
|
||||
# `fnm use` |
||||
|
||||
``` |
||||
fnm-use 1.24.0 |
||||
Change Node.js version |
||||
|
||||
USAGE: |
||||
fnm use [FLAGS] [OPTIONS] [version] |
||||
|
||||
FLAGS: |
||||
-h, --help Prints help information |
||||
--install-if-missing Install the version if it isn't installed yet |
||||
-V, --version Prints version information |
||||
|
||||
OPTIONS: |
||||
--arch <arch> |
||||
Override the architecture of the installed Node binary. Defaults to arch of fnm binary [env: FNM_ARCH] |
||||
[default: x64] |
||||
--fnm-dir <base-dir> The root directory of fnm installations [env: FNM_DIR] |
||||
--log-level <log-level> The log level of fnm commands [env: FNM_LOGLEVEL] [default: info] |
||||
--node-dist-mirror <node-dist-mirror> |
||||
https://nodejs.org/dist/ mirror [env: FNM_NODE_DIST_MIRROR] [default: https://nodejs.org/dist] |
||||
|
||||
|
||||
ARGS: |
||||
<version> |
||||
``` |
Loading…
Reference in new issue